The simplest solution to prevent the form submission is to return false on submit event handler defined using the onsubmit property in the HTML
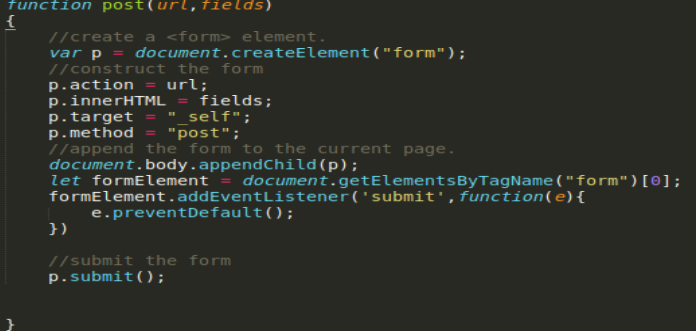
The simplest solution to prevent the form submission is to return false on submit event handler defined using the onsubmit property in the HTML