Are you ready to delve into the world of Windows Management Instrumentation (WMI) using C#? WMI opens up a plethora of possibilities for accessing system resources and retrieving vital information about your Windows environment. In this comprehensive guide, we’ll walk through the process of retrieving all the services running on your system using the powerful C# programming language.
Understanding WMI: A Brief Overview
WMI, short for Windows Management Instrumentation, serves as the gateway for .NET Framework applications to interact with system resources. It provides a rich set of classes tailored for querying system information, monitoring performance, and even managing system settings. Think of it as your window into the inner workings of your Windows operating system.
Getting Started: Creating a Windows Application
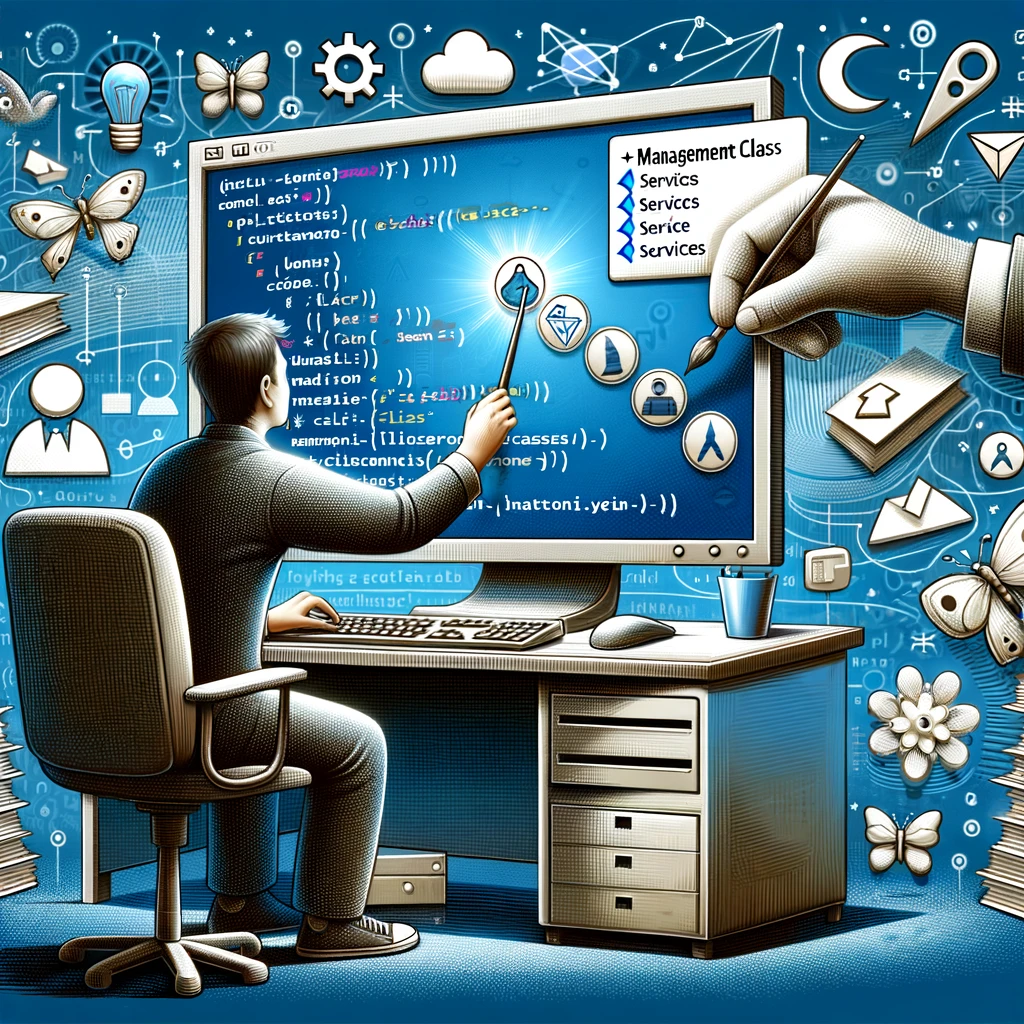
Let’s kick off our journey by creating a Windows application in C#. Follow these simple steps to set up your development environment:
Step | Description |
---|---|
Create a New Project | Fire up your preferred IDE and create a new Visual C# Windows Application project. |
Design the User Interface | Design your form with essential controls like a ListBox and a Button. Customize as needed. |
Add Event Handlers | Double-click the button to create a click event handler for retrieving services. |
Include System.Management Reference | Add a reference to System.Management.dll to gain access to WMI functionality. |
Also read:What is a kahoot?
Code Implementation: Retrieving Services
Now, let’s dive into the exciting part – writing the code to retrieve all the services running on your system. Below is a breakdown of the code snippets involved:
- Adding Required Namespace:
// Sample 01: Required Namespace
using System.Management;
- Creating Management Class:
// Sample 02: Create Management Class
ManagementClass service_class = new ManagementClass("Win32_Service");
- Retrieving Service Instances:
// Sample 03: Get all the Objects of Win32_Service class
ManagementObjectCollection Service_objects_collection = service_class.GetInstances();
- Iterating Through Services:
// Sample 04: Iterate through each service and display its name
foreach (ManagementObject service in Service_objects_collection)
{
ctrlLstBox.Items.Add(service["Name"].ToString());
}
Putting It All Together: The Complete Code
Here’s the complete code for your reference:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Management;
namespace CreateInstance{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnServices_Click(object sender, EventArgs e)
{
// Create Management Class
ManagementClass service_class = new ManagementClass(“Win32_Service”);
// Get all the Objects of Win32_Service class
ManagementObjectCollection Service_objects_collection = service_class.GetInstances();
// Iterate through each service and display its name
foreach (ManagementObject service in Service_objects_collection)
{
ctrlLstBox.Items.Add(service[“Name”].ToString());
}
}
}
}
Conclusion
Congratulations! You’ve successfully learned how to harness the power of WMI in C# to retrieve all the services running on your Windows system. With this newfound knowledge, you can explore endless possibilities for system administration, monitoring, and automation.
Now, go ahead and experiment with WMI to unlock even more insights into your Windows environment!
Also read: Fix Physical Memory Dump Error on Windows
Questions and Answers
- What is WMI?
- WMI stands for Windows Management Instrumentation, a powerful framework for accessing and managing system resources in Windows.
- What can I do with WMI?
- With WMI, you can query system information, monitor performance, manage system settings, and much more.
- How do I get started with WMI in C#?
- Simply include the
System.Management
namespace in your C# project and start exploring the rich set of classes provided by WMI.
- Simply include the
- Can I use WMI for remote system management?
- Yes, WMI supports remote management, allowing you to interact with system resources on remote computers.
- Is WMI only available for Windows?
- Yes, WMI is a Windows-specific technology and is not available on other operating systems.
- What are some common use cases for WMI?
- Common use cases include system monitoring, inventory management, software deployment, and configuration management.
- Are there any performance considerations when using WMI?
- While WMI provides powerful capabilities, excessive use can impact system performance, so it’s essential to use it judiciously.
- Can I use WMI to manage Active Directory?
- Yes, WMI provides classes for managing Active Directory objects, allowing you to automate administrative tasks.
- How do I handle errors when using WMI?
- WMI provides error handling mechanisms to help you gracefully handle exceptions and errors that may occur during operations.
- Where can I find more resources on WMI?
- There are plenty of online resources, tutorials, and documentation available to further your understanding of WMI and its capabilities.
Also read: Connection failed: Too many connections